Exploring C# 10: Use Extended Property Patterns to Easily Access Nested Properties
Welcome back to my series on new C# 10 features. So far we've talked about file-scoped namespaces and global using declarations. Today, we'll talk about extended property patterns in C# 10.
Over the last few years, C# has made a lot of property pattern enhancements. Starting with C# 8, the language has supported extended property patterns, a way to match an object's specific properties.
For our example, let's continue the superhero theme with a Person
, a Superhero
that inherits from Person
, and a Suit
. We're going to calculate any special surcharges when making a particular superhero's suit.
public class Person
{
public string? FirstName { get; set; }
public string? LastName { get; set; }
public string? Address { get; set; }
public string? City { get; set; }
}
public class Superhero : Person
{
public int MaxSpeed { get; set; }
public Suit? Suit { get; set; }
}
public class Suit
{
public string? Color { get; set; }
public bool HasCape { get; set; }
public string WordsToPrint { get; set; }
}
Now let's create a quick object for Iron Man:
var tonyStark = new Superhero()
{
FirstName = "Tony",
LastName = "Stark",
Address = "10880 Malibu Point",
City = "Malibu",
Suit = new Suit
{
Color = "Red",
HasCape = false
}
};
In our switch expression, let's say we want to determine special pricing based on a particular suit's color.
Here's how we'd do it in C# 9:
public static int CalculateSuitSurcharge(Superhero hero) =>
hero switch
{
{ Suit: { Color: "Blue" } } => 100,
{ Suit: { Color: "Yellow" } } => 200,
{ Suit: { Color: "Red" } } => 300
_ => 0
};
With C# 10, we can use dot notation to make it a bit cleaner:
public static int CalculateSuitSurcharge(Superhero hero) =>
hero switch
{
{ Suit.Color: "Blue" } => 100,
{ Suit.Color: "Yellow" } => 200,
{ Suit.Color: "Red" } => 300
_ => 0
};
Much like before, you can also use multiple expressions at once. Let's say we wanted to charge a surcharge for a notoriously difficult customer. Look at how we can combine multiple properties in a single line.
public static int CalculateSuitSurcharge(Superhero hero) =>
hero switch
{
{ Suit.Color: "Blue" } => 100,
{ Suit.Color: "Yellow" } => 200,
{ Suit.Color: "Red", Address: "10880 Malibu Point" } => 500,
{ Suit.Color: "Red" } => 300
_ => 0
};
That's all there is to it. Will this feature change your life? No. Even so, it's a nice update that allows for cleaner and simpler code.
There's a lot more you can do with property patterns as well, so I'd recommend hitting up the Microsoft Docs to learn more.

If you're interested in the design proposal, you can also find it below.
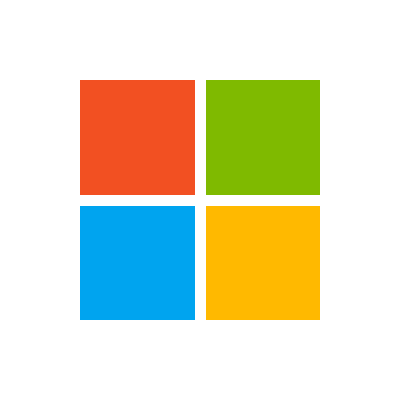
Let me know your thoughts below. Happy coding!